Vue Js Dropdown change color on Select : In Vue.js, you can change the colour of a dropdown option when it is selected by binding a style attribute to the option element and using a computed property or a method to set the desired colour and background colour based on the option’s selected status. For example, you can create a method property called “changeColor” that returns a CSS class with the desired colour when the option is selected and bind this property to the option’s class attribute. Then, when the option is selected, the selectedClass method property will update and apply the appropriate colour to the option.
How do I change the color of a dropdown option ?
Here’s an example of how you can change the colour of the selected option in a drop-down menu using Vue.js: In this example, we have a select
element that is bound to a selected
data property using v-model
. We also have an options
data property that contains an array of options for the drop-down menu. Each option has a colour
property that is initially set to the default colour that we want the option to be when it’s not selected. In the changeColor
method, we loop through the options and check the value of the selected option against the value
property of each option. If the values match, we set the colour of that option to yellow and the background colour to black. If not, we set the colour to initial
so that it goes back to the colour that was set initially.
Vue Js change the color of a dropdown option example
<select v-model="selectedOption" style="width:250px" @change="changeColor">
<option disabled value="">Please select one</option>
<option v-for="option in options" :value="option.language"
:style="{ color: option.color,backgroundColor:option.bgColor }">
{{option.language}}</option>
</select>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
selectedOption: '',
options: [
{ id: 1, language: 'React', },
{ id: 2, language: 'Vue', },
{ id: 3, language: 'Angular' },
{ id: 4, language: 'Node' },
{ id: 5, language: 'Express' },
{ id: 6, language: 'Bootstrap' },
{ id: 7, language: 'MongoDb' },
]
}
},
methods: {
changeColor() {
this.options.forEach(option => {
if (option.language === this.selectedOption) {
option.color = 'yellow';
option.bgColor = 'black'
}
else {
option.color = 'initial'
option.bgColor = 'initial'
}
}
)
}
}
});
</script>
Output of above example
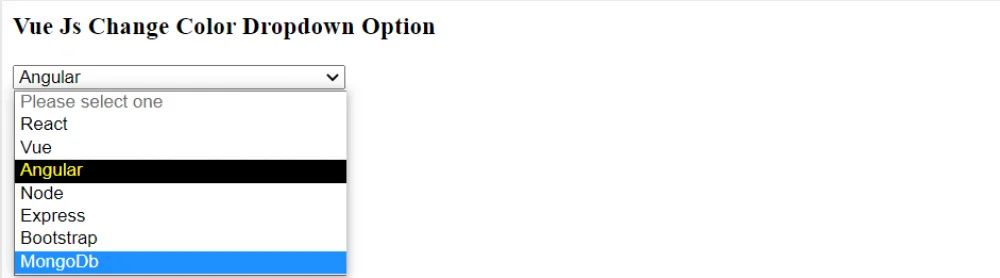